Building RESTful APIs with Node.js: A Step-by-Step Tutorial on Creating Robust APIs Using Node.js and Express
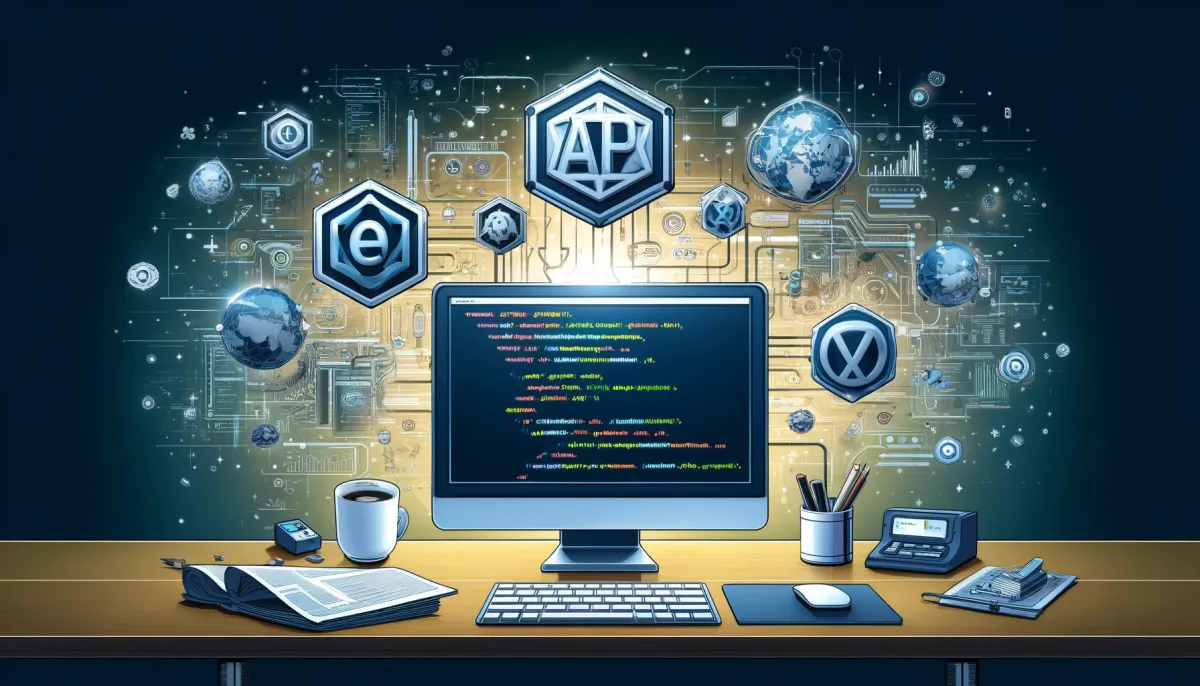
In today's digital world, APIs (Application Programming Interfaces) are essential. They allow different software systems to communicate with each other, enabling the integration of various services and the building of complex applications. Among the many ways to create APIs, building RESTful APIs with Node.js and Express is one of the most popular due to its simplicity and efficiency. In this blog post, we will guide you through the process of creating robust RESTful APIs using Node.js and Express.
What is a RESTful API?
A RESTful API is an application program interface (API) that uses HTTP requests to GET, PUT, POST, and DELETE data. REST (Representational State Transfer) is a set of architectural principles that makes network communication more scalable and manageable. RESTful APIs adhere to these principles, making them easy to use and understand.
Why Node.js and Express?
Node.js is a runtime environment that allows you to execute JavaScript on the server side. It is known for its non-blocking, event-driven architecture, making it efficient and scalable. Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
Prerequisites
Before we dive in, make sure you have the following installed:
- Node.js
- npm (Node Package Manager)
You can download and install Node.js and npm from the official website.
Step 1: Setting Up the Project
First, create a new directory for your project and navigate into it using the terminal:
bashCopy codemkdir
my-rest-apicd
my-rest-api
Initialize a new Node.js project:
bashCopy codenpm init -y
This command creates a package.json
file with default settings.
Step 2: Installing Dependencies
Next, we need to install Express and other necessary packages:
bashCopy codenpm install express body-parser mongoose
express
: A web framework for Node.js.body-parser
: Middleware to parse incoming request bodies.mongoose
: A MongoDB object modeling tool designed to work in an asynchronous environment.
Step 3: Creating the Server
Create a new file called server.js
and add the following code:
const express = require('express();
());
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
app.use(bodyParser.jsonapp.listen(port, () =>{
console.log(`Server is running on http://localhost:${port}`);
});
This code sets up a basic Express server that listens on port 3000.
Step 4: Connecting to MongoDB
For this tutorial, we will use MongoDB as our database. Make sure you have MongoDB installed and running on your machine. You can download it from the official MongoDB website.
Create a new file called db.js
and add the following code:
const mongoose = require('mongoose');
const connectDB = async () => {
try {
await mongoose.connect('mongodb://localhost:27017/mydb'{,
useNewUrlParser: true,
useUnifiedTopology: true
});
console.log('MongoDB connected...');
} catch(err) { console.error(err.message);
process.exit(1);
}
};
module.exports = connectDB;
In server.js
, require the db.js
file and call the connectDB
function to connect to MongoDB:
const connectDB = require('./db');
connectDB();
Step 5: Defining a Model
Create a new directory called models
and inside it, create a file called Item.js
:
const mongoose = require('mongoose');
const ItemSchema = new mongoose.Schema({
name: {
type: String,
required: true
},
quantity: {
type: Number,
required: true
},
date: {
type: Date,
default: Date.now
}
});
module.exports = mongoose.model('Item', ItemSchema);
This code defines a simple model for an item with name
, quantity
, and date
fields.
Step 6: Creating Routes
Create a new directory called routes
and inside it, create a file called items.js
:
const express = require('express');
const router = express.Router();
const Item = require('../models/Item');
// @route GET api/items
// @desc Get all items
// @access Public
router.get('/', async(req, res) => { try{
const items = await Item.find();
res.json(items);
} catch(err) { console.error(err.message
); res.status(500).send('Server Error');
}
});
// @route POST api/items
// @desc Create an item
// @access Public
router.post('/', async(req, res) => { const { name, quantity } = req.body;
();
try {
let item = new Item({
name,
quantity
});
item = await item.save res.json
(item); } catch
(err) { console.error(err.message
); res.status(500).send('Server Error');
}
});
// @route DELETE api/items/:id
// @desc Delete an item
// @access Public
router.delete('/:id', async(req, res) => { try{
await Item.findByIdAndRemove(req.params.id);
res.json({ msg: 'Item removed'}); } catch(err) {
console.error(err.message);
res.status(500).send('Server Error');
}
});
module.exports = router;
This code defines routes for getting all items, creating an item, and deleting an item.
Step 7: Integrating Routes
In server.js
, integrate the routes by adding the following code:
const items = require('./routes/items');
app.use('/api/items', items);
Step 8: Testing the API
Start the server by running the following command:
bashCopy codenode server.js
You can test the API using Postman or any other API testing tool. The available endpoints are:
GET /api/items
: Get all items.POST /api/items
: Create a new item.DELETE /api/items/:id
: Delete an item by ID.
Conclusion
In this tutorial, we have covered the basics of building a RESTful API using Node.js and Express. We set up a project, connected to a MongoDB database, defined a model, created routes, and tested the API. This is just the beginning; you can extend this API by adding more features, such as user authentication, data validation, and more.
Building RESTful APIs with Node.js and Express is a powerful way to create scalable and maintainable applications. With the knowledge gained from this tutorial, you can start building your own APIs and integrate them into your projects.
Happy coding!