Exploring Lodash: A Comprehensive Guide to the Essential npm Package
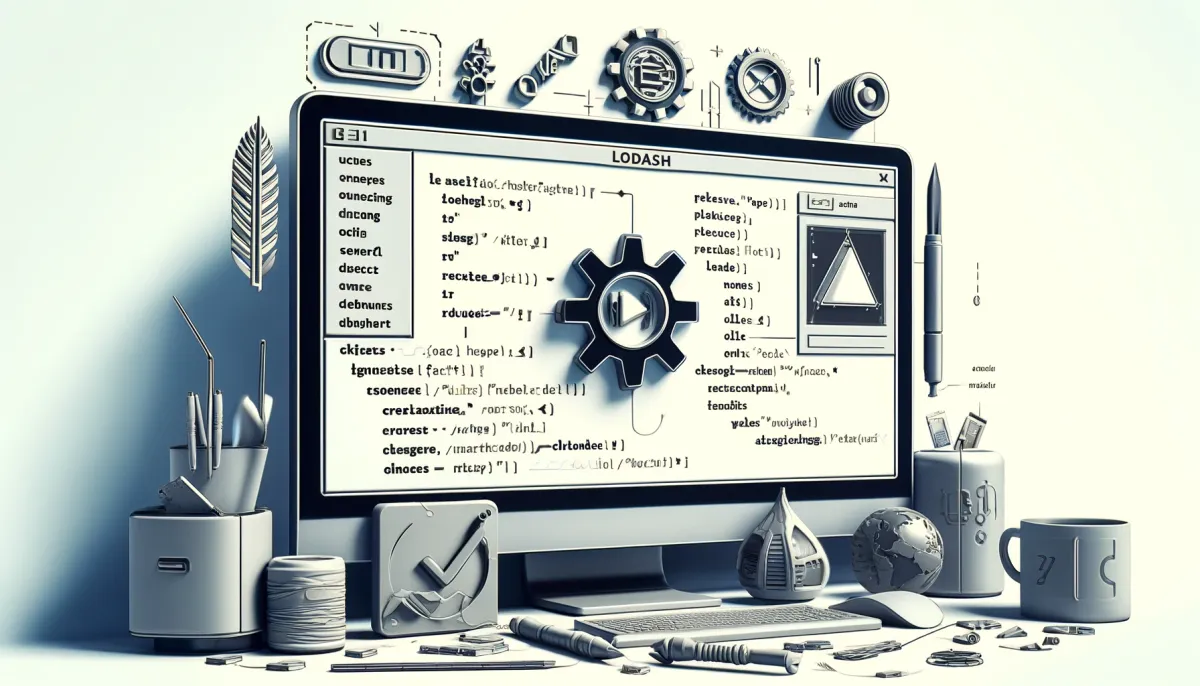
Introduction
In the vast ecosystem of JavaScript libraries and frameworks, certain tools stand out due to their efficiency, versatility, and widespread adoption. Lodash is one such library that has become a staple for many developers. This comprehensive guide will delve into what Lodash is, why it is so widely used, and how you can leverage its powerful functions to enhance your JavaScript projects.
What is Lodash?
Lodash is a modern JavaScript utility library that provides a wide range of functions to simplify common programming tasks. It is designed to make it easier to work with arrays, numbers, objects, strings, and more. The library is built with performance in mind, offering methods that are both efficient and easy to use.
Key Features of Lodash
- Collection Manipulation: Lodash offers a plethora of functions for manipulating arrays and objects. These include methods for filtering, mapping, reducing, and more.
- String Manipulation: The library includes various functions for working with strings, such as trimming, padding, and case conversion.
- Function Utilities: Lodash provides utilities for working with functions, including debouncing, throttling, and binding.
- Object Utilities: Functions for merging, cloning, and comparing objects are also part of the package.
- Template Rendering: Lodash includes a simple and powerful templating engine for rendering HTML templates.
Why Use Lodash?
1. Simplicity and Readability
Lodash simplifies many complex operations, making code more readable and maintainable. For example, chaining methods allows for concise and expressive code.
2. Performance
Lodash is optimized for performance. The functions are implemented in a way that minimizes memory usage and execution time.
3. Consistency
Using Lodash ensures consistent behavior across different browsers and environments. This cross-platform compatibility is crucial for many projects.
How to Install Lodash
Installing Lodash is straightforward using npm (Node Package Manager). You can add it to your project by running the following command:
bashCopy codenpm install lodash
Once installed, you can import the library in your JavaScript files:
const _ = require('lodash');
Or, if you are using ES6 modules:
import _ from 'lodash';
Commonly Used Lodash Functions
1. _.map
The _.map
function creates a new array by applying a function to each element in the input array.
const numbers = [1, 2, 3];
const doubled = _.map(numbers, (num) => num * 2);
console.log(doubled); // [2, 4, 6]
2. _.filter
The _.filter
function creates a new array with all elements that pass a test implemented by the provided function.
const numbers = [1, 2, 3, 4, 5];
const evens = _.filter(numbers, (num) => num % 2 === 0);
console.log(evens); // [2, 4]
3. _.reduce
The _.reduce
function reduces an array to a single value by executing a reducer function on each element of the array.
const numbers = [1, 2, 3, 4, 5];
const sum = _.reduce(numbers, (total, num) => total + num, 0);
console.log(sum); // 15
4. _.cloneDeep
The _.cloneDeep
function creates a deep copy of a value. This is useful for creating a copy of an object or array that does not share references with the original.
const obj = { a: 1, b: { c: 2}};
const clone = _.cloneDeep(obj);
console.log(clone); // { a: 1, b: { c: 2 } }
Advanced Usage
1. Chaining
Lodash supports chaining, allowing you to chain multiple methods together for more complex operations.
const numbers = [1, 2, 3, 4, 5];
const result = _(numbers)
.map((num) => num * 2)
.filter((num) => num > 5)
.value();
console.log(result); // [6, 8, 10]
2. Custom Builds
To optimize performance, you can create custom builds of Lodash that include only the functions you need. This reduces the bundle size and improves load times.
bashCopy codenpm install lodash-cli -g
lodash include=map,filter
Conclusion
Lodash is an indispensable tool for JavaScript developers, offering a wide range of functions that simplify and streamline common programming tasks. Its efficiency, versatility, and ease of use make it a go-to library for many projects. Whether you are manipulating arrays, strings, objects, or functions, Lodash provides the utilities needed to make your code cleaner, faster, and more maintainable.
By incorporating Lodash into your workflow, you can save time and effort, allowing you to focus on building robust and scalable applications. Happy coding!