Getting Started with Node.js: A Comprehensive Guide for Beginners
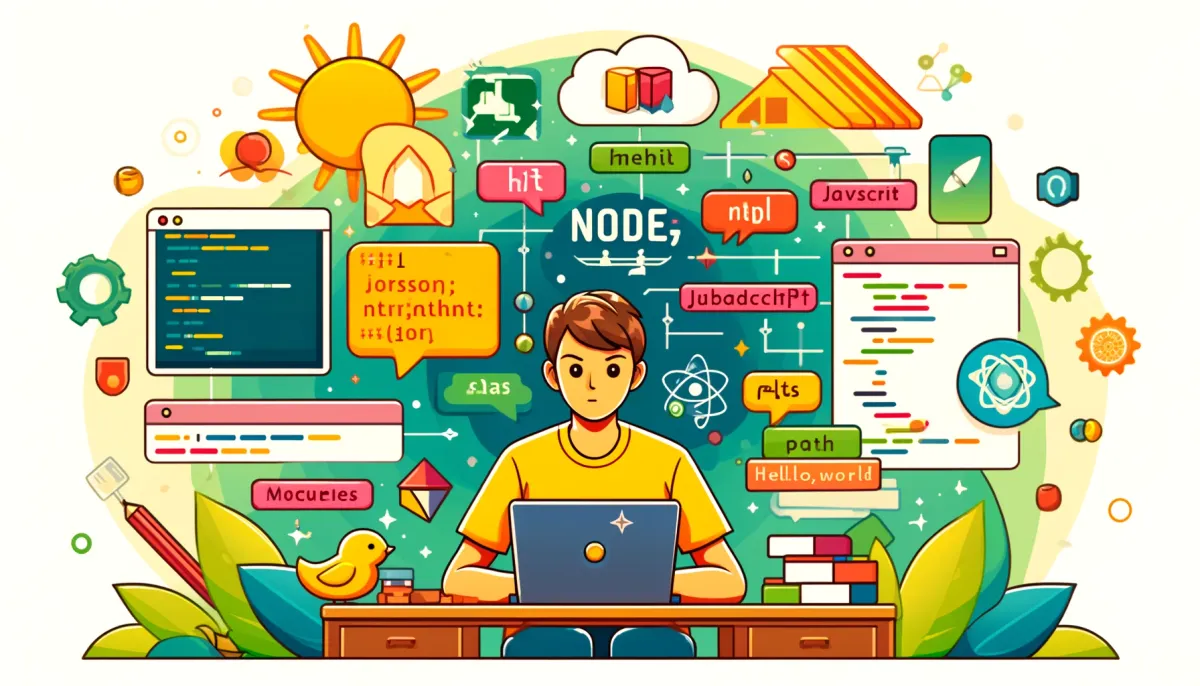
Introduction
Node.js has become a cornerstone of modern web development, revolutionizing how we build server-side applications. Whether you're new to programming or transitioning from another language, Node.js offers a powerful and flexible environment for developing scalable and high-performance applications. This guide aims to provide a comprehensive introduction to Node.js, helping you get started with your first Node.js project.
What is Node.js?
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. It allows developers to execute JavaScript code outside of a web browser, making it possible to build server-side and networking applications. Node.js is event-driven and non-blocking, which makes it ideal for building real-time applications that require high throughput.
Why Choose Node.js?
- JavaScript Everywhere: With Node.js, you can use JavaScript for both client-side and server-side development, simplifying the development process and allowing code reuse.
- High Performance: Node.js's non-blocking, event-driven architecture ensures efficient handling of multiple concurrent connections, making it perfect for real-time applications.
- Rich Ecosystem: The Node.js ecosystem, powered by npm (Node Package Manager), offers a vast collection of libraries and tools to extend the functionality of your applications.
- Active Community: A vibrant and active community supports Node.js, constantly contributing to its improvement and providing a wealth of resources for learning and troubleshooting.
Setting Up Your Development Environment
- Install Node.js: Start by downloading and installing Node.js from the official website. The installer will also include npm, which is essential for managing your project dependencies.
- Choose a Code Editor: Select a code editor that suits your needs. Popular choices include Visual Studio Code, Sublime Text, and Atom.
Verify Installation: Open your terminal or command prompt and type the following commands to verify the installation:
node -v
npm -v
These commands should return the installed versions of Node.js and npm, respectively.
Creating Your First Node.js Application
- Create an Entry Point: Create a new file named
app.js
in your project directory. This file will serve as the entry point for your application.
Run Your Application: In the terminal, navigate to your project directory and run:
node app.js
Open your web browser and go to http://127.0.0.1:3000/
to see your application in action.
Write Your First Script: Open app.js
and add the following code:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Initialize a Project: Navigate to your project directory and run the following command to create a new Node.js project:
npm init
This command will prompt you to enter details about your project and generate a package.json
file, which manages your project's dependencies and metadata.
Exploring Node.js Modules
Node.js has a rich set of built-in modules that you can use to perform various tasks. Some of the most commonly used modules include:
- HTTP: Create HTTP servers and clients.
- FS (File System): Interact with the file system.
- Path: Handle and transform file paths.
- Events: Work with event-driven programming.
You can also install third-party modules from npm to extend the functionality of your application. For example, you can use the express
module to create robust web servers or the mongoose
module to interact with MongoDB databases.
Building a Simple Web Server with Express
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. Here’s how to create a simple web server using Express:
Run Your Express Server: In the terminal, run:
node app.js
Open your web browser and go to http://localhost:3000/
to see your Express application in action.
Update app.js
: Replace the content of app.js
with the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
Install Express: Run the following command to install Express:
npm install express
Conclusion
Getting started with Node.js is an exciting journey into the world of server-side JavaScript development. With its powerful features, extensive ecosystem, and active community, Node.js is an excellent choice for building scalable and high-performance applications. By following this guide, you've taken your first steps towards becoming a proficient Node.js developer. Keep exploring, experimenting, and building, and soon you'll be creating amazing applications with Node.js.